Bitmap2LCD is a tool for programming small Graphic LCDs in embedded systems.
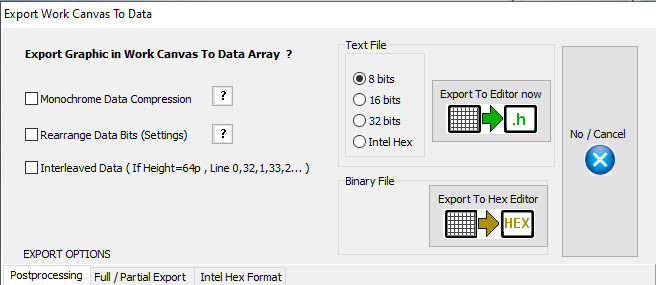
Update V3.9H
A simple data compression feature, for the output of GLCD data arrays is implemented in Bitmap2LCD
This function is only available for monochrome mode and 8 bit output format.
The 8 bit microcontrollers for price sensitive projects are circuits with often less onchip memory space than most of the 16 or 32 bit devices.
The target is to save as many as microcontroller flash memory as possible. As tables for full display patterns of for example a 128 x 64 dot matrix LCD need 1024 bytes each, the goal of this function was to save flash space for more code or graphics or just to reduce the overall flash capacity and therefore to sink the price of the MCU chip.
The microcontroller firmware has to be able to handle these tables with a special code, which decodes the compressed data. Processing time for decompression has to be allowed.
How does it work ?
Instead of only converting the black and white pixels found in the work canvas to a linear list of n bytes, with the data compression method explained here, the data array is split into two separate arrays in one single output file : one as usual for the data stream and another for the pointers of each data groups.
The basic concept of this compression is based on making groups of consecutive identical data bytes in the data array.
Consecutive identical byte chains, and consecutive different byte chains are handled, the goal here is to save data bytes when a consecutive identical byte chain is found. While the first pointer of a consecutive different bytes chain is a loss of one data byte stored as a pointer, a consecutive identical byte chain of 10 bytes is a win of 8 bytes, the data being written only once in the data array. The count of them is then stored in the pointer byte array part.
The maximum count of consecutive data, identical or different is limited to 127 ( pointer bits 6 to 0 ). If a data count in a group reaches 127, a new group is encoded.
The bit 7 of the pointer byte is the flag for the compression decoder. A 1 (high) is for a chain of identical data bytes, and a 0 (low) is for an chain of different data.
When data compression is active, Bitmap2LCD converts all data and shows the compression rate in the compression statistics at the end of the output file.
This method of compression shows different results in vertical or horizontal orientation conversion, it depends of the LCD graphic !
If possible, before to choose the LCD controller and its specific data orientation in Display RAM , you could try both orientations and compare the possible compression ratios.
In the Header Include file, the example script below shows how to setup the compression table information.
( It is an example for a GNU-C compiler for ATMEL AVR family )
Everything after the tag [&COMPRESSION] is a script information for the data compression function.
The tag [&CNAME] is replaced in the output table name, by the data array name
with an additional suffix _x ( For example : Newfile_x )
The tag [&CSIZE] is not used yet (v2.3)
In the Header Include file :
[&COMPRESSION]
const prog_uint8_t [&CNAME][&CSIZE] = {
Please also check the online forum for other topics about this function
Compression Decoder Example
The below example of a function in C language, decodes compressed data arrays converted with bitmap2LCD.
It is for an Atmel AVR target MCU with GCC compiler, a T6963C LCD controller ( horizontal byte orientation from left to right )
No buffer RAM.
Note : For LCD module widths greater than 255 dots, x and width variables should be long integers